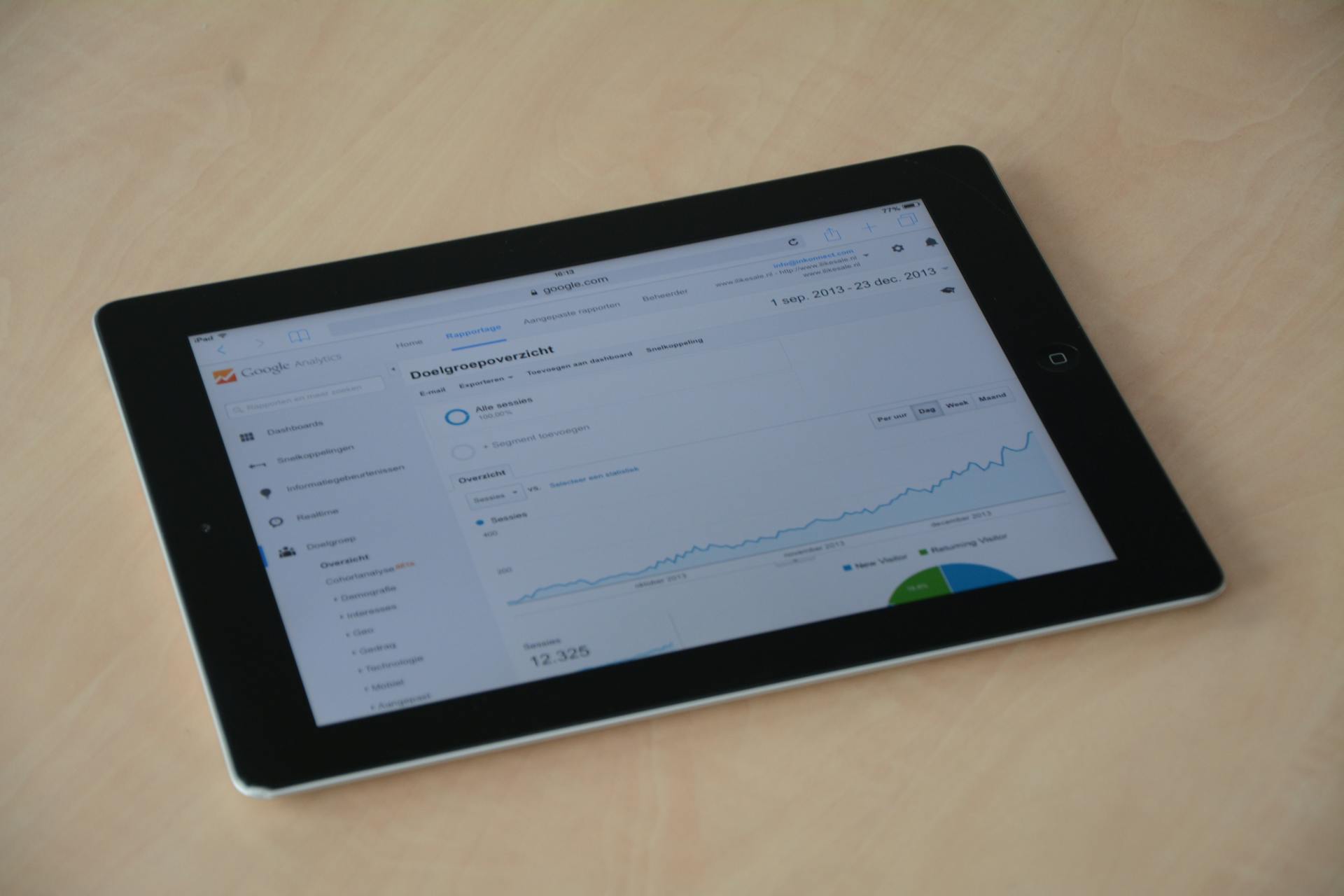
As a Nextjs developer, you're likely familiar with the importance of tracking user behavior and analytics to improve your application's performance. Nextjs provides built-in support for analytics through its API routes.
To get started, you'll need to install the analytics package, which can be done using npm or yarn. This will allow you to collect data on page views, unique visitors, and other key metrics.
The Nextjs analytics API route is a simple way to track events and page views, and it's easy to integrate with popular analytics services like Google Analytics. You can also use the API route to track custom events, such as button clicks or form submissions.
Setting Up Posthog
To set up Posthog on the client side, you'll need to create a providers.js file and set up the PostHogProvider with the 'use client' directive. This allows Posthog to work with Next.js' app router.
The 'use client' directive is crucial because Next.js assumes your page is server-side rendered without it, causing errors with client hooks like usePostHog.
Once you've set up the providers.js file, import it in your app/layout.js file and wrap your app in the PostHog provider.
This will enable autocaptured events into your PostHog instance and allow you to use PostHog on the client side in all your client-side rendered Next.js components.
If you don't use the 'use client' directive, you'll need to use the PostHog Node SDK to interact with PostHog on the server side.
Tracking and Events
Tracking and events are essential for any Next.js application, and LogSnag provides a range of tools to help you do so. You can track custom events on the client-side using the `posthog.capture()` method, or on the server-side using the PostHog Node SDK.
To track events on the client-side, you can use the `trackEvent` function, which takes in parameters such as `category`, `action`, `name`, `value`, and `dimensions`. For example, you can track a custom event like this: `posthog.capture('Custom Event', 'Category', 'Action', 'Name', 10, { dimension: 'value' })`.
Alternatively, you can use the `useLogSnag` hook to track events programmatically. This is useful when you need to track an event based on a specific condition. You can also use the `trackContentInteraction` function to track manual content interaction events, or the `trackContentInteractionNode` function to track interaction with a block in DOM node.
Client Side Pageviews
To capture pageviews on the client side, you'll need to use the app router in Next.js. This involves adding code to your provider.js file.
Next.js acts as a single-page app, so you'll need a new implementation for capturing pageviews beyond the first-page load. You can do this by creating a separate file named PostHogPageView.jsx.
In this file, you'll use the usePathname and useSearchParams client-side hooks from next/navigation to check when the URL changes and capture a custom $pageview event. You'll also use the data from the pathname and search params to accurately create a string for the $current_url property.
To avoid the initial pageview from being duplicated, you'll need to set capture_pageview: false in the posthog.init call in app/providers.js. This ensures that you only capture pageview events for individual pages.
Events with UseLogSnag Hook
You can use the useLogSnag hook to track events programmatically. This hook allows you to track events in your Next.js application with ease.
To use the useLogSnag hook, you can pass in the category, action, and name of the event as arguments. For example, you can track a custom event like this: `useLogSnag.trackEvent('category', 'action', 'name')`.
Here's a breakdown of the trackEvent method:
You can also use the useLogSnag hook to track page views automatically. Simply call the `trackPageView` method like this: `useLogSnag.trackPageView('customPageTitle')`.
Additionally, you can use the `trackContentInteraction` method to track manual content interaction events. This method takes four arguments: `contentInteraction`, `contentName`, `contentPiece`, and `contentTarget`.
You can also use the `trackContentInteractionNode` method to track interaction with a block in a DOM node. This method takes two arguments: `domNode` and `contentInteraction`.
Customization and Configuration
Customization and Configuration is key to making the most out of your Next.js project's analytics capabilities. You can store your Google Analytics Measurement ID in a secure and easily configurable environment file.
To do this, you'll create a .env.local file in the root of your project, where you can store your environment variables, including your Measurement ID. This file will serve as the central hub for your project's environment variables.
By using the NEXT_PUBLIC_GA_MEASUREMENT_ID environment variable, you can keep your Measurement ID secure and easily changeable without modifying your code. This is a much more elegant solution than hardcoding your Measurement ID directly into your code.
Using Data Attributes
Using data attributes is a great way to track events in your Next.js application. You can simply add the data-event attribute to any element in your application, and LogSnag will automatically track the event when the element is clicked.
There are optional attributes you can use to customize the event, including data-channel, which specifies the channel to which the event should be sent, defaulting to events. You can also use data-icon to specify an emoji icon for the event, such as :rocket:. Additionally, you can use data-tag-name to add custom tags to the event.
Here are some examples of how to use data attributes to track events:
By using data attributes, you can easily track events in your Next.js application without writing any code. This is a great way to get started with tracking events and can be a good alternative to using the useLogSnag hook or the PostHogClient.
Set Link Timer
Setting a link timer can be a lifesaver when it comes to tracking events. The JavaScript Tracking Client will lock the page for a fraction of a second to give the request time to reach the Collecting & Processing Pipeline.
This feature is particularly useful when visitors quickly close the page after producing an event, such as when opening a link. The request might get cancelled, but the timer prevents this from happening.
You can set the link tracking timer using the setLinkTrackingTimer function, which takes a single argument: the time in seconds. This function is a simple way to ensure that your tracking requests don't get lost in the shuffle.
Configuring Environment Variables
To keep your Google Analytics Measurement ID secure and easily configurable, store it in an environment file. Create a .env.local file in the root of your Next.js project to store your environment variables, including your Google Analytics Measurement ID.
Next.js automatically loads environment variables defined in the .env.local file. You can access these variables in your components or pages using process.env.
To set up Google Analytics, import the Script component in your _app.js file and use your environment variable to set up the tracking code. This ensures that your Google Analytics tracking code is loaded asynchronously, improving your website's performance.
By using the NEXT_PUBLIC_GA_MEASUREMENT_ID environment variable, you keep your Measurement ID secure and easily changeable without modifying your code.
Server-Side and Advanced Topics
Server-rendered components are the default for the app router, which means we must use the PostHog Node SDK to get server-side rendered feature flags or other data from PostHog.
To set this up, create a posthog.js file in the app folder that returns a PostHog Node client, which can then be used to get access to the Node client in our server components. This allows us to get the basics of PostHog set up on both the client and server side with Next.js and the app router.
Error
Error tracking is a crucial aspect of monitoring and debugging your application.
You can attempt to send error tracking requests using the trackError function, which follows the same format as native errors caught by enableJSErrorTracking.
This function will only send error requests when JS error tracking is enabled, so make sure to call enableJSErrorTracking before attempting to track errors.
The trackError function will also respect rules for tracking only unique errors, so you won't be flooded with duplicate error reports.
Error tracking can be a lifesaver when debugging complex issues, and using trackError can help you get to the root of the problem.
PostHog with Server-Rendered Components
Server-rendered components are the default for the app router.
If we want server-side rendered feature flags or get other data from PostHog, we must use the PostHog Node SDK.
To set this up, create a posthog.js file in the app folder that returns a PostHog Node client.
This function can then be used to get access to the Node client in our server components.
With this, you have the basics of PostHog set up on both the client and server side with Next.js and the app router.
Server-Side Events
Server-side events can be a powerful tool for tracking user activity in your application. You can use a server-side client to track events, such as when a user signs up.
The server-side client should only be used in server-side code, so you'll need to separate your tracking code from your client-side code. This is a good practice to follow to keep your code organized.
You won't have access to the user ID set in the client-side code, so you'll need to set it manually. This might require some additional code to fetch the user ID from your database or other storage solution.
You can use the server-side client to track a wide range of events, from user sign-ups to more complex interactions. Just be sure to follow the guidelines for using the server-side client.
Sources
- https://posthog.com/tutorials/nextjs-app-directory-analytics
- https://webmasters.stackexchange.com/questions/142132/implementing-google-analytics-in-next-js
- https://developers.piwik.pro/en/latest/data_collection/web/frameworks/Piwik_PRO_Library_for_NextJS.html
- https://logsnag.com/blog/nextjs-product-analytics
- https://www.dhiwise.com/post/integrating-nextjs-google-analytics-a-step-by-step-guide
Featured Images: pexels.com