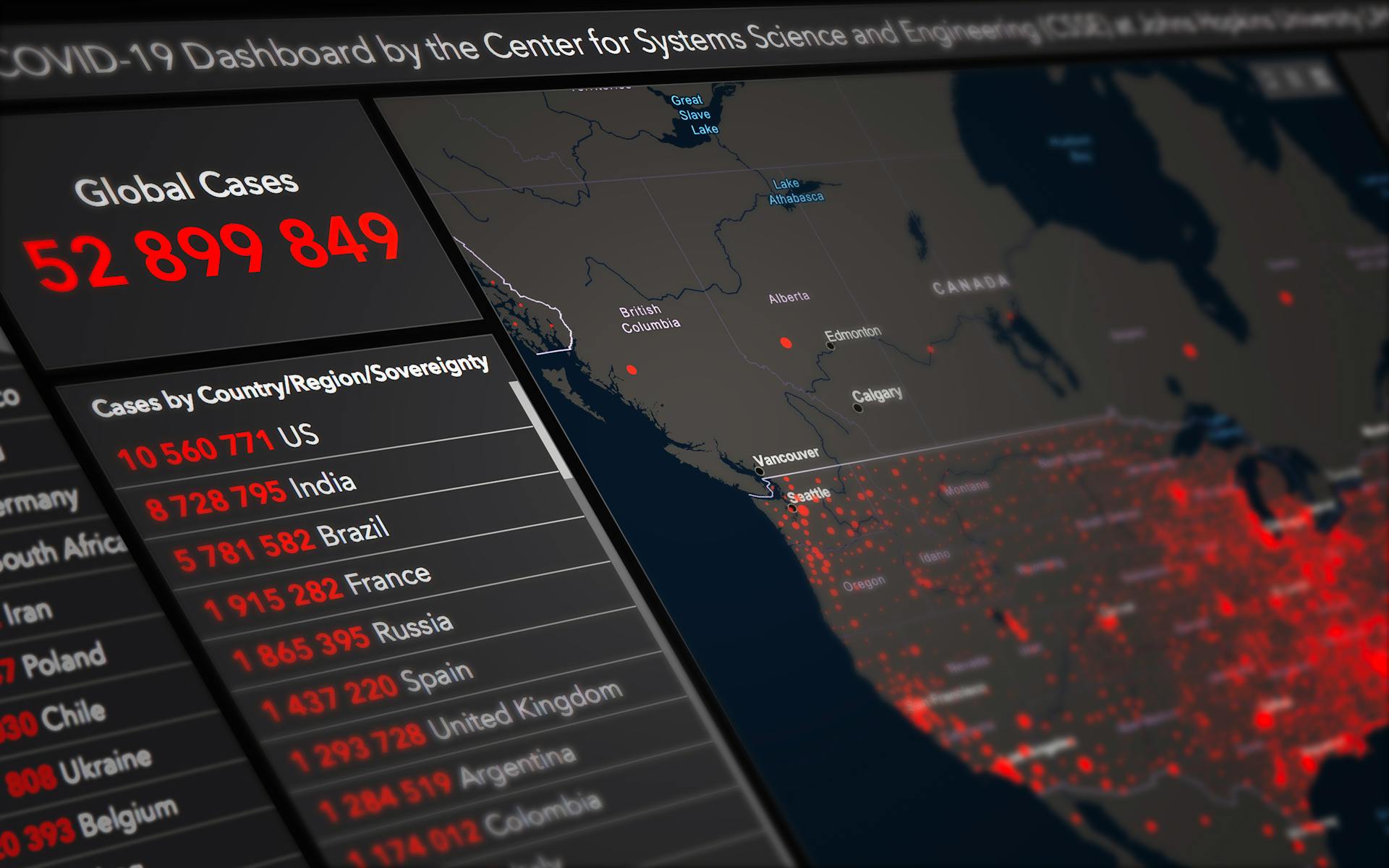
Setting up a new Next.js project with GraphQL is as simple as running `npx create-next-app my-app --experimental-app` and then installing the `@apollo/client` package.
With Next.js, you can create API routes using the `api` directory, which is a great place to start building your GraphQL API.
To start building your GraphQL schema, you'll need to create a new file in the `pages/api` directory, such as `index.js`.
By using the `graphql` tag in your API route, you can define your GraphQL schema and start building your API.
Consider reading: Next Js Cookie
Setting Up Next.js with GraphQL
To set up Next.js with GraphQL, you can start by creating a new app with Create Next App. This will give you a solid foundation to work with.
The project structure is crucial, so let's get that right. The api folder is where your API or server lives, and since you'll be using GraphQL, you'll need a resolver and a schema to create a GraphQL server.
For more insights, see: Next Js Project
The endpoint of the server will be accessible on the path /api/graphql, which is the entry point of the GraphQL server. This is where the magic happens.
To use Apollo Server within Next.js, you'll need to install apollo-server-micro. This will enable you to leverage the power of GraphQL in your Next.js app.
To request data from the Github API, you'll need to install the axios library. This will allow you to fetch data from the API and use it in your GraphQL schema.
Here are the key steps to setting up Next.js with GraphQL:
- Create a new app with Create Next App.
- Set up the project structure with an api folder for your GraphQL server.
- Install apollo-server-micro to use Apollo Server within Next.js.
- Install axios to request data from the Github API.
By following these steps, you'll be well on your way to setting up Next.js with GraphQL and unlocking the full potential of your app.
Creating GraphQL Schema
Creating a GraphQL schema is the first step in building a GraphQL server.
A GraphQL schema defines the shape of your data graph.
In a GraphQL schema, you can define types that describe the shape of your data. For example, in the code snippet, a User type is defined with an id of type ID, a login, and an avatar_url of type String.
Consider reading: Nextjs App Route Get Ssr Data
This User type is then used in the getUsers query that has to return an array of users, and the getUser query that fetches a single user with the name of the user as a parameter.
With a GraphQL schema created, you can now update the resolver file to generate responses from GraphQL queries.
Related reading: Query in Nextjs
Create the Schemas
A GraphQL schema defines the shape of your data graph. This is crucial for understanding how your data will be structured and queried.
To create a new GraphQL schema, you'll need to define the types of data you're working with. In the case of a Github user, you might define a User type that expects an id of type ID, a login, and an avatar_url of type String.
A GraphQL schema can also include queries to fetch data. For example, you can define a getUsers query that returns an array of users, or a getUser query that fetches a single user by name.
Defining a solid content model is also essential when creating a GraphQL schema. This involves defining the structure of your data, such as a Page with a slug field.
When querying data with Union Types, you'll need to use the ... on TypeName notation to query each of your models. This allows you to fetch data from multiple sources in a single query.
Create the Resolvers
A GraphQL resolver is a set of functions that allows you to generate a response from a GraphQL query.
To create the resolvers, you'll need to add some meaningful code to the resolvers file, specifically in api/resolvers/index.js.
The resolvers file matches the queries name defined earlier on the GraphQL Schema with the resolver functions.
The getUsers function enables you to retrieve all users from the API and then return an array of users that needs to mirror the User type.
You use the getUser method to fetch a single user with the help of the name passed in as a parameter.
With this in place, you now have a GraphQL Schema and a GraphQL resolver, it's time to combine them and build up the GraphQL Server.
Related reading: Nextjs Server Actions File Upload
Fetching Inside Components
You can fetch data inside components in Next.js using the fetch API, but it's more efficient to use Apollo Client. With Server Components, you can await the result of your data fetching inside your component, making it easier to deal with data fetching.
One thing to note is that in Server Components, you're not allowed to use any hooks, including ApolloProvider and useQuery. But don't worry, you can still use the client directly in your components.
Here are some ways to fetch data inside components:
- Using the fetch API directly
- Using Apollo Client with the @apollo/experimental-nextjs-app-support library
- Creating a custom hook for fetching data
To use Apollo Client with Server Components, you can install the @apollo/experimental-nextjs-app-support library and use the registerApolloClient function to get the Apollo Client. Then, you can use the client directly in your Server Component.
Here's an example of how to use Apollo Client with Server Components:
- Create a Server Component and use the Apollo Client to fetch data
- Use the useSuspenseQuery hook to fetch data in a Client Component
- Create a custom hook for fetching data and use it in your components
Remember to use the "use client" directive in your Client Components to allow for streaming SSR rendering.
Related reading: Nextjs Use Server
API Routes and Form Handling
Next.js API Routes allow you to extend your Next.js App by adding a backend to it. This is a powerful feature that enables you to build APIs with Node.js, Express, and GraphQL.
To handle forms in Next.js, you can use API Routes to process form data on the server-side. This means you can create a file inside the pages/api folder and use it as an API endpoint to handle form submissions.
API Routes in Next.js use the file-system to treat files inside the pages/api folder as API endpoints. This allows you to access your API endpoint on a URL like https://localhost:3000/api/your-file-name.
By using Next.js API Routes for form handling, you can keep your front-end and back-end code organized and separate. This is a key benefit of using Next.js for building APIs and back-end functionality.
Check this out: Nextjs Usecontext
Cache
Next.js caches all fetch requests, including those made with Apollo Client, regardless of whether they're GET or POST requests.
This means that if you're using Apollo Client, you'll want to update your client to avoid caching on the server-side. We can do this by passing fetch options to our queries, such as revalidating them every 5 seconds.
Apollo Client allows us to pass fetch options to our queries using the context argument. We can also use Segment Options to specify caching options for a particular page.
By revalidating our GraphQL queries, we can ensure that the data is up-to-date and not cached forever. This is especially important for pages that require real-time data.
The InMemoryCache is the default cache implementation for Apollo Client, which stores GraphQL data in a normalized, in-memory, JSON object. This approach allows for quick retrieval and updating of data, enhancing performance and responsiveness.
We can customize the Apollo cache to suit our needs by defining type policies and custom merge functions to handle specific fields differently. This ensures that data-fetching operations are optimized and managed effectively, reducing the need for redundant network requests.
Worth a look: Using State in Next Js
Testing and Advanced Usage
To test your Next.js GraphQL API, you can use the built-in `graphql` tag in your pages to fetch data from the API. This allows you to see how the data is being fetched and rendered in the browser.
For more advanced usage, you can use the `useQuery` hook from `@apollo/client` to fetch data from the API in your components. This hook can be used to fetch data in a more declarative way, making it easier to manage complex queries.
Using the `useQuery` hook, you can also handle errors and loading states in a more robust way, making your application more resilient to failures and slow network connections.
Curious to learn more? Check out: Nextjs Loading
Test the
Testing the GraphQL server is crucial to ensure it's working as expected. We can fetch a single user with a query, and our server works as expected.
To test the server, we can use a query to retrieve a single user. Great! Our server works as expected.
The GraphQL server can be tested with a query to fetch a single user.
Worth a look: Nextjs Spa
Advanced Usage
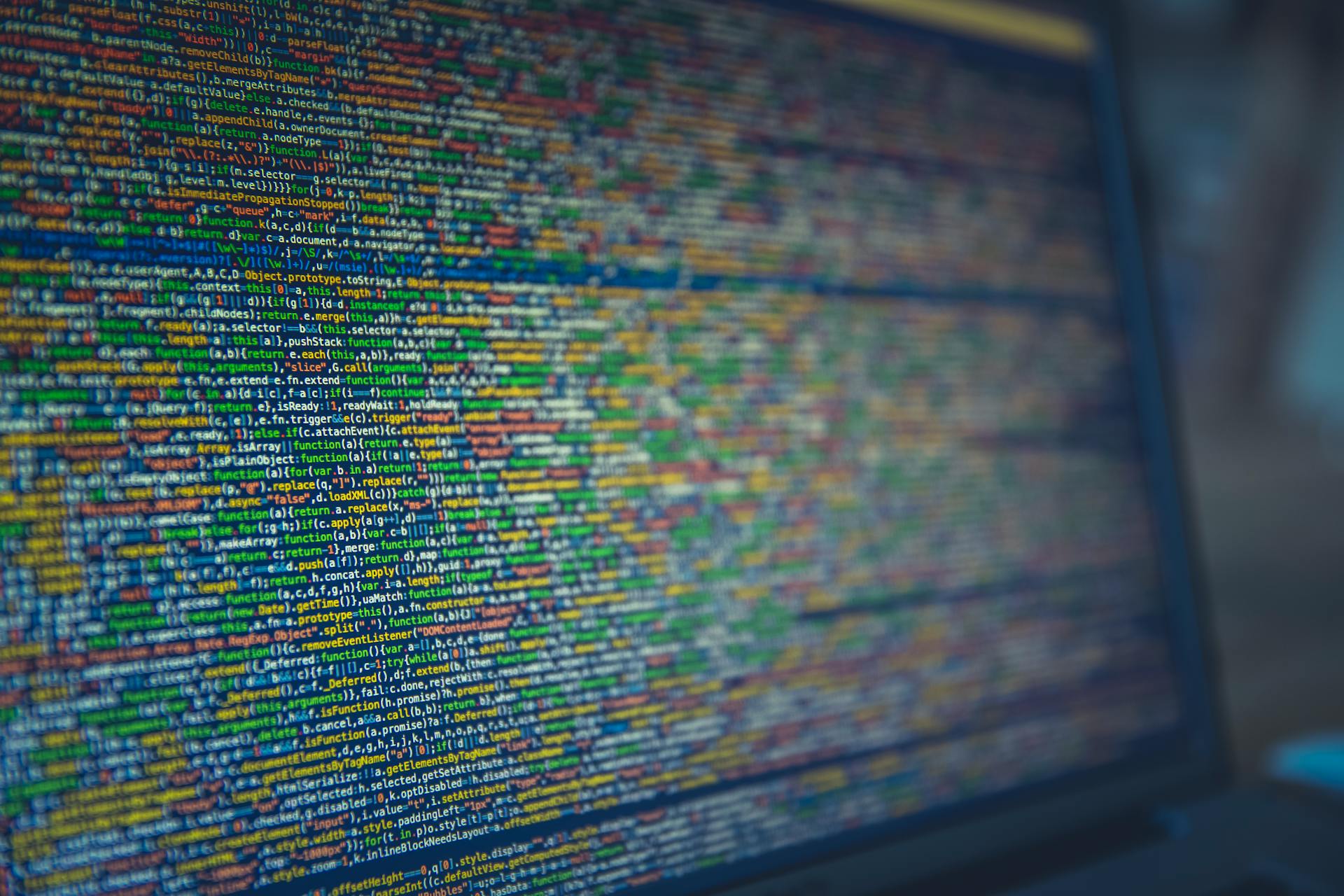
The advanced usage of testing tools is where things get really interesting. You can create custom test cases by combining individual test steps in various ways.
For example, you can use the "OR" operator to add multiple conditions to a single test step. This is especially useful when you're testing for multiple possible outcomes.
Test data can be easily imported and exported using the tool's built-in CSV file functionality.
This feature is super handy for reusing test data across different test cases.
You can also use the tool's API to automate testing tasks and integrate it with other tools and systems.
This opens up a whole new world of possibilities for automating testing tasks and streamlining your testing process.
Consider reading: Use Client Nextjs
Components and Rendering
In Next.js, all pages inside the app directory are Server Components by default, but you can use the "use client" directive to tell React that a component is a client component, which means it will be rendered both on the server side and on the client side.
Expand your knowledge: Next Js Client Side Rendering
To use Apollo Client with Next.js, you can use the @apollo/experimental-nextjs-app-support library, which allows you to use the useSuspenseQuery hook for streaming SSR rendering. This hook is used in conjunction with the "use client" directive.
You can create an ApolloWrapper component that does all the setup for you, including passing the client to the hooks. This wrapper component can be used in your layout file, making sure that all pages using this layout have access to the Apollo Client.
To implement client-side rendering with Apollo Client, you can use the useQuery hook to fetch data when the component mounts. This approach is straightforward but may impact initial load performance.
Server-side rendering with Apollo Client can be implemented in Next.js using getServerSideProps, which fetches the data on the server before sending the HTML to the client. This can improve the initial load time and is beneficial for SEO.
React Server Components (RSC) allow you to render parts of your React application on the server, improving performance and user experience. To use Apollo Client with RSC, you can create a Server Component that fetches and renders data on the server, delivering fully rendered HTML to the client.
Intriguing read: Next Js React Fundamentals
Here's a summary of the different approaches:
Note that each approach has its own trade-offs and use cases, and you should choose the one that best fits your needs.
Network Request Features
Apollo Client offers advanced network request features that can further optimize your data fetching strategy.
You can use the Apollo Link Batching feature to batch multiple GraphQL operations into a single HTTP request, reducing the number of network requests and improving performance.
This can be especially useful for complex applications with multiple queries.
Batching Requests can be achieved by utilizing the Apollo Link Batching feature.
Here are the two main features of Apollo Link Batching:
- Batches multiple GraphQL operations into a single HTTP request
- Reduces the number of network requests
Additionally, Apollo Client provides experimental Suspense support for seamless integration with React Suspense, which can improve loading state management and user experience.
This allows for a more seamless and efficient data fetching experience.
Suspense Support is a great feature for developers looking to improve their application's performance and user experience.
See what others are reading: Nextjs Suspense
Frequently Asked Questions
What is the difference between next JS GraphQL and REST?
Next.js with GraphQL offers more flexibility and control for complex data needs, while Next.js with REST provides simplicity and ease of use for straightforward projects
Sources
- https://www.apollographql.com/blog/how-to-use-apollo-client-with-next-js-13
- https://www.smashingmagazine.com/2020/10/graphql-server-next-javascript-api-routes/
- https://hygraph.com/blog/nextjs-graphql
- https://hygraph.com/blog/forms-and-submissions-with-nextjs-and-graphql
- https://www.dhiwise.com/post/a-comprehensive-guide-to-nextjs-apollo-client-integration
Featured Images: pexels.com