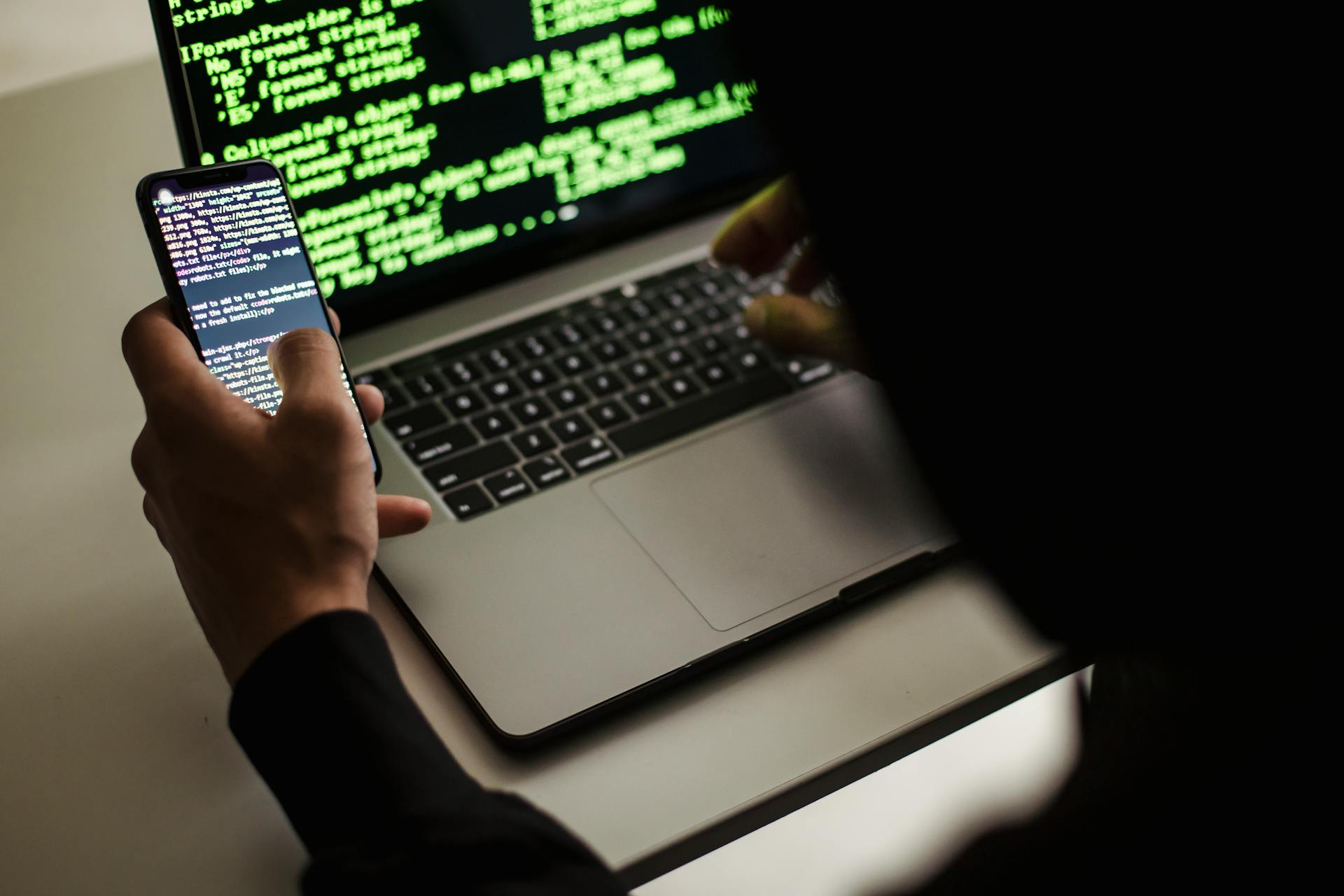
So you're prepping for a Next.js interview? Well, you've come to the right place! Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized web applications.
Next.js is built on top of React, so having a solid understanding of React concepts is essential. You should be familiar with React components, state management, and lifecycle methods.
To get started, make sure you're comfortable with the basics of Next.js, such as routing, pages, and API routes. You can check out the official Next.js documentation for a comprehensive overview.
Understanding the differences between client-side and server-side rendering is also crucial. Server-side rendering allows for better SEO and faster page loads, while client-side rendering is ideal for complex applications with a lot of dynamic content.
Broaden your view: Nextjs Pathname Server Component
Next.js Fundamentals
To ace a Next.js Framework interview, you should focus on key skills that can be evaluated in a single session. These core competencies are particularly important to assess during the interview phase.
Next.js Framework skills that are crucial to evaluate include assessing every aspect of a candidate's expertise, which is challenging to do in a single interview. This requires focusing on key skills that can provide valuable insights into the candidate's abilities.
Focusing on these key skills can help you get a better understanding of the candidate's Next.js expertise and make a more informed hiring decision.
For your interest: Next.js
SSR, SSG, and CSR Comparison
SSR (Server-Side Rendering) in Next.js generates HTML for a page on the server for each request, making it useful for dynamic content that changes frequently.
SSR improves performance and SEO by rendering content on the server rather than in the browser. This approach is particularly beneficial for content-heavy websites or pages that require up-to-date data for each request.
Static Site Generation (SSG) pre-renders pages at build time, creating static HTML files that can be served quickly. SSG is ideal for pages with content that doesn't change often.
For another approach, see: Nextjs Rendering
Client-Side Rendering (CSR) loads a minimal HTML shell and uses JavaScript to render content on the client side after the initial load. CSR is great for highly interactive applications where the initial content isn't SEO-critical.
The choice between SSR and CSR depends on factors such as SEO requirements or performance considerations. SSR is suitable for dynamic content, while CSR is better for interactive applications.
To implement dynamic routes with SSR in Next.js, you can use the file-based routing system and implement getServerSideProps for server-side rendering. This approach provides SEO and performance benefits for dynamic pages.
getStaticProps is used to fetch data at build time and pre-render the page with the fetched data, making it suitable for static sites. getServerSideProps, on the other hand, is used to fetch data on each request at server-side before rendering the page, making it suitable for dynamic content.
Next.js provides server-side rendering and static site generation out of the box, making it easier to create SEO-friendly and optimized web pages. It comes with features like automatic code splitting, API routes, and pre-rendering, which enhance performance and user experience.
The getStaticProps function in Next.js is used for static site generation to fetch data at build time. It allows you to fetch data from an API, database, or any other source and pass it as props to your page component.
A unique perspective: What Is Ssr in Nextjs
Handling Errors and Error Pages
You can manage errors in Next.js both globally and at the page level. This gives you flexibility in how you handle errors.
To create a custom error page for specific HTTP error codes like 404 or 500, simply add a 404.js or 500.js file inside the /pages directory. Next.js will automatically use these files to render the appropriate error pages.
You can also use try-catch blocks within your data fetching methods like getServerSideProps or getStaticProps to catch errors and handle them accordingly. This might involve setting a redirect or passing an error state to the page component.
Creating a custom _error.js page in the /pages directory provides a fallback for any unhandled errors. This allows you to show a custom error message or perform logging.
To provide even more robust error handling, consider combining these methods with React's error boundaries. This will help you manage errors both during server-side rendering and on the client side.
Explore further: Next Js Local Font
Skills to Evaluate
Evaluating a candidate's Next.js skills can be a daunting task, but focusing on key skills can provide valuable insights.
You should evaluate their understanding of Next.js core competencies, particularly those mentioned in Next.js Framework interviews.
A mix of practical tests and targeted questions helps find the right fit for your team, streamlining your hiring process.
Start with a Next.js skills test to gauge candidates' practical knowledge, which quickly identifies top performers.
Use targeted questions from the interview to dive deeper into their Next.js expertise and problem-solving abilities.
Accessing Next.js tests and front-end developer assessments can help you find your next star developer faster and more accurately.
What Is Next.config.js?
The next.config.js file is a crucial part of a Next.js project, allowing you to customize the default configuration settings of your application.
It enables you to set environment variables, customize webpack configurations, and set up redirects and rewrites. You can also use it to enable experimental features.
Customizing your Next.js application with next.config.js makes it an essential part of optimizing and configuring your app to suit your specific needs.
Related reading: Can Nextjs Be Used on Traditional Web Application
Link Component Role
The Link component in Next.js is used for client-side navigation between pages of the app.
This approach leverages the power of the Next.js router to perform navigation dynamically, resulting in faster transitions.
Instead of triggering a full page reload, the Link component fetches only the necessary data to display the next page, reducing load times.
As a result, this setup preserves state and provides a smoother user experience.
Explore further: Nextjs Link
Building and Deploying
When building a Next.js application, you typically start by optimizing it for production using the command next build. This step is crucial for performance.
You can then deploy your application to various hosting providers, including Vercel, which offers seamless integration and is a popular choice. Simply connect your repository to Vercel, and it will manage the rest, including automatic builds and deployments on new commits.
Deploying to other platforms like AWS, Heroku, or DigitalOcean requires using Docker to containerize your app or setting up build and start scripts. Many hosting providers also offer guides specifically for Next.js apps to help streamline the process.
Worth a look: Auth Provider Nextjs
Deploying an Application
To deploy a Next.js application, you'll typically start by building it for production using the command next build. This optimizes your app for the best performance.
You can then deploy it to various hosting providers, such as Vercel, which offers seamless integration and automatic builds and deployments on new commits. Vercel is a popular choice for deployment because it's the company behind Next.js.
Alternatively, you can deploy to platforms like AWS, Heroku, or DigitalOcean, which might require using Docker to containerize your app or setting up the build and start scripts manually. The command next start will launch your application in production mode after you've built it.
Many hosting providers offer guides specifically for Next.js apps that can help streamline the process.
Common deployment strategies for Next.js applications include deploying to a platform-as-a-service (PaaS) provider like Vercel or Netlify. This involves using their services to host and manage your app.
You can also set up continuous integration and continuous deployment (CI/CD) pipelines with tools like GitHub Actions or Jenkins. This ensures that your app is automatically built and deployed whenever changes are made to the codebase.
Consider reading: Can Vercel Host Nextjs Servers
Customizing 404 Pages
Customizing 404 pages is an essential part of creating a great user experience in Next.js. You can create a custom 404 page by adding a 404.js file in the pages directory.
This file will automatically be used to render any non-existent routes, allowing you to customize the look and feel of your 404 error page. You can also use static generation or server-side rendering methods within this component if needed.
A well-designed 404 page should provide helpful navigation links or a search bar to guide users back to valid content. This is especially important for user experience.
To provide an even better experience, consider adding a clear and concise error message that explains what went wrong and how the user can resolve the issue. This will help users feel more in control and less frustrated.
By following these tips, you can create a custom 404 page that not only looks great but also helps users find what they're looking for. This is a great opportunity to showcase your brand's personality and style.
Curious to learn more? Check out: Nextjs 404 Page
Performance Optimization
To optimize performance in a Next.js app, start by utilizing built-in features like server-side rendering and static site generation to optimize loading times for different types of content.
Next.js automatically handles code splitting, which only loads the JavaScript necessary for the page being viewed, helping with faster page loads.
Implementing API routes can handle backend logic within your app, minimizing the need for an external server and reducing latency.
Use Lighthouse or similar tools to regularly audit your app's performance and pinpoint any bottlenecks, whether they're related to large assets, redundant code, or inefficient data fetching.
Shallow routing allows you to change the URL without running data fetching methods like getStaticProps or getServerSideProps again, resulting in improved performance and a smoother user experience.
By enabling shallow routing, you can update the URL for state changes or navigation purposes without re-fetching data, making it a useful feature for applications where data fetching is not necessary.
If this caught your attention, see: Next Js Api Call
Code splitting, image optimization, and automatic static optimization are just a few of the built-in optimizations provided by Next.js, which can help optimize your app's performance.
Static site generation and server-side rendering are also built-in features that allow you to choose how you want to fetch and render data depending on your app's performance needs.
Next.js also offers automatic static optimization, which figures out which pages can be served as static assets and pre-renders them at build time, resulting in super-fast loading times for those pages.
To optimize performance in Next.js, use techniques such as code splitting, image optimization, and server-side rendering, which can help reduce bundle size, improve loading speeds, and enhance user experience.
Consider reading: Next Js 14 Redirect to Another Page Loading Indicator
Internationalization and Localization
Next.js has a built-in internationalization feature that makes it easy to create multi-language applications. This feature is called i18n routing.
To enable i18n routing, you need to specify the locales and default locale in the next.config.js file. This configuration allows automatic locale detection and routing.
For more insights, see: Next Js Localization
You can also use a library like next-i18next to handle internationalization. This library provides a configuration file called i18n.js where you specify your locales, namespaces, and backend configurations.
The next-i18next library also provides an appWithTranslation HOC and a useTranslation hook to access translation functions within your components. This makes it easy to serve localized content to users.
Ideal candidates should be able to explain how Next.js simplifies the process of internationalization and localization. They should mention the configuration in next.config.js and the use of hooks or components for navigation.
See what others are reading: Nextjs Useeffect
Security Considerations for Using a
Security is a top priority when building web applications with Next.js, and there are several key considerations to keep in mind.
Cross-site scripting (XSS) and cross-site request forgery (CSRF) are two common security vulnerabilities that Next.js developers should be aware of. To prevent XSS, make sure to properly sanitize any user-generated content and use features like Next.js's built-in next/script component to safely inject scripts.
CSRF can be mitigated by using tokens to secure your API routes. Regularly updating your dependencies is also crucial to avoid known vulnerabilities. Next.js provides features like built-in CSRF protection and content security policies to help developers secure their projects.
To ensure the security of a Next.js project, implement built-in security features like CSRF protection and content security policies. These features provide automatic XSS protection and help prevent common security vulnerabilities.
Be mindful of using environment variables, as exposing sensitive information on the client side can be a major security risk. Use API routes or server-side logic to handle sensitive operations instead.
Recommended read: Nextjs 14 New Features
API Routes and Integration
API routes in Next.js allow you to build your own API endpoints within your application, and they live in the pages/api directory. To create one, you simply add a JavaScript or TypeScript file in that directory, and the file name will correspond to the endpoint path.
See what others are reading: Can Amazon S3 Take in Nextjs File
Common use cases for API routes in a Next.js application include handling form submissions, fetching data from external APIs, interacting with databases, and performing authentication tasks. These routes provide a way to build server-side functionality without needing a separate backend server, simplifying the project structure and deployment process.
To integrate APIs with a Next.js project, you can use tools like fetch or third-party libraries to fetch data from APIs. This is a common requirement in modern web applications, and Next.js provides built-in support for fetching data from APIs.
You might enjoy: Nextjs Fetch
What Are API Routes?
API routes in Next.js are serverless functions that allow you to create backend logic and data fetching within your application.
They live in the pages/api directory, where you can add JavaScript or TypeScript files to create endpoints. The file name corresponds to the endpoint path, so if you create a file called hello.js, you can access that API endpoint via /api/hello.
See what others are reading: Nextjs File Upload Api
To create an API route, you export a default function that takes req and res parameters, the request and response objects. You can then use these to handle different HTTP methods and send back responses.
For example, if you create a file called hello.js, you can export a function that responds with a JSON message when a GET request is made. If any other HTTP method is used, it will return a 405 status code.
API routes in Next.js are a powerful tool for building backend logic and data fetching within your application, and they're easy to create and use.
Additional reading: How to Use Reducer Api in Next Js 14
API Route Use Cases
API routes in Next.js are used to create backend endpoints within a Next.js application.
They simplify the project structure and deployment process by allowing you to build server-side functionality without needing a separate backend server.
One common use case for API routes is handling form submissions, which can be a complex task that requires server-side processing.
Intriguing read: Is Next Js Frontend or Backend
API routes can also be used to fetch data from external APIs, interact with databases, and perform authentication tasks.
By using API routes, you can keep your application architecture simple and maintainable, even when dealing with complex backend operations.
API routes provide a flexible way to handle server-side operations and integrate with external services, making them a powerful tool in your Next.js toolbox.
In practice, API routes can be used to handle tasks like user registration, password reset, and payment processing, which require secure and reliable server-side processing.
By leveraging API routes, you can create a robust and scalable application architecture that meets the needs of your users.
A different take: Next Js Aws Amplify Gen2 Architecture Diagram
Integrating APIs with a Project
API integration is a common requirement in modern web applications, and Next.js provides built-in support for fetching data from APIs. You can integrate APIs with a Next.js project using tools like fetch or third-party libraries.
To integrate APIs effectively, candidates should be able to explain how to handle API requests and responses. This includes understanding how to use tools like fetch and third-party libraries to make API requests.
Expand your knowledge: Next Js Fetch Data save in Context and Next Route
API integration is crucial for building full-stack applications, and Next.js allows creating API routes to facilitate this process. By creating API routes, you can build server-side functionality without needing a separate backend server.
To create an API route, you simply create a file inside the "pages/api" directory with the desired endpoint and write your server-side logic using Node.js. This allows you to handle form submissions, fetch data from external APIs, interact with databases, and perform authentication tasks.
In Next.js, you can handle data fetching using the `getStaticProps` or `getServerSideProps` functions. `getStaticProps` fetches data at build time and is ideal for static pages, while `getServerSideProps` fetches data on every request and is useful for dynamic content or when data frequently changes.
Next.js provides several methods for route-based data fetching, including `getStaticProps`, `getServerSideProps`, and `getStaticPaths`. These methods allow you to fetch data at build time or request time, depending on the specific needs of your application.
By using API routes and integrating APIs with your project, you can simplify the project structure and deployment process, and build a more robust and scalable application.
Here's an interesting read: Routes in Nextjs
What Is the Fallback Property in GetStaticPaths?
The fallback property in getStaticPaths is used to handle paths that weren't generated during the build time. It's a game-changer for scenarios where you have a large number of dynamic paths and can't realistically generate all of them at build time.
By setting fallback to true, Next.js will render the page on-demand and cache it for subsequent requests. This means users will still get the content they're looking for, even if it wasn't pre-rendered.
If you set fallback to false, any request for a path not returned by getStaticPaths will result in a 404 page. This is a drastic measure, but it ensures that users will know the page doesn't exist.
There's also a blocking option, which serves content immediately, putting the client on hold until the generated page is ready. This option balances the need for instant gratification with the need for page rendering to complete.
Intriguing read: Framer Motion Page Transitions Nextjs Examples
State Management and Middleware
State management in Next.js is approached in several ways, depending on the complexity of your app. For simpler state management, you can use React's built-in useState and useEffect hooks, which work seamlessly with Next.js since it's built on React.
For more complex state management needs, you might want to leverage libraries like Redux, Context API, or even Zustand. These solutions help manage state that's shared across multiple components or pages.
Middleware plays a crucial role in handling requests and responses in a Next.js project, allowing you to run code before a request is completed and acting as a bridge that can intercept requests and responses. You can create a middleware by adding a _middleware.js file in the pages or API folder.
See what others are reading: Nextjs Middleware Matcher
Implementing Dynamic
You can implement dynamic routing in Next.js by using file name conventions inside the pages directory. Create a file with square brackets to indicate a dynamic segment, such as [id].js inside pages/posts/. This will catch dynamic ids, like /posts/1 or /posts/some-slug.
Inside that dynamic file, use Next.js's useRouter hook from next/router to access the dynamic part of the URL. For example, const router = useRouter(); const { id } = router.query; will give you the id when you visit /posts/1.
You might enjoy: Next Js File Upload
Dynamic routing in Next.js allows for creating dynamic routes based on the parameters provided in the URL. This flexibility is perfect for generating pages based on data fetching or other dynamic content.
To fetch data for your dynamic routes, you can use getStaticPaths and getStaticProps for static generation or getServerSideProps for server-side rendering. getStaticPaths allows you to define a list of paths that need to be pre-rendered, and getStaticProps lets you fetch the data for each page at build time.
You can define complex dynamic routes by using nested dynamic routes, like pages/posts/[postId]/[commentId].js. This will allow you to access multiple dynamic segments in the URL.
Recommended read: Next Js Get Current Url
Middleware Importance
Middleware plays a crucial role in handling requests and responses in a Next.js project.
Middleware allows you to run code before a request is completed, acting as a bridge that can intercept requests and responses. It's like having a mini layer where you can handle things like authentication, logging, or modifying the request and response objects before they hit your main handler or get sent back to the client.
If this caught your attention, see: Nextjs 13 Middleware
You can create a middleware by adding a _middleware.js file in the pages or API folder. This file can define a function that receives req and res objects, giving you the power to execute custom logic.
Middleware is a powerful tool for managing behavior across multiple pages without duplicating code. It lets you check if a user is logged in before allowing access to certain routes, for example.
A different take: Iron Session Next Js Middleware
Managing State
You can manage state in a Next.js application using React's built-in useState and useEffect hooks for simpler state management needs. These hooks work seamlessly with Next.js and are sufficient for component-specific state like form inputs or UI toggles.
For more complex state management needs, libraries like Redux, Context API, or Zustand can be leveraged. Redux offers a predictable state container and works well with Next.js through the next-redux-wrapper package.
To manage state that's shared across multiple components or pages, consider using Redux or the Context API. The Context API is a great option for medium-sized apps where a more lightweight solution is needed compared to Redux.
Take a look at this: Redux with Nextjs
Next.js provides support for Server-Side Rendering (SSR) and Static Site Generation (SSG) methods like getServerSideProps and getStaticProps. These functions can fetch and pre-render data on the server, ensuring that your state is populated before your component renders.
You can use getServerSideProps to fetch data on the server and pre-render it for the client. This approach ensures that your state is populated before your component renders, making it ideal for data that needs to be available on both the client and the server.
For another approach, see: Nextjs Ui Library
Head Component Usage
The Head component in Next.js is used to modify the head section of your HTML document, allowing you to customize elements like the title, meta tags, and link tags on a per-page basis.
This is particularly useful for setting meta tags for SEO, adding stylesheet links, or inserting any other element that would usually go inside the tag of an HTML document.
You can import Head from 'next/head' and then wrap your head elements within the Head component inside your component's render method.
Each page can have its own unique head content, making your application more flexible and enhancing its SEO performance.
Expand your knowledge: Next Js Head
Testing and Evaluation
When evaluating a candidate's Next.js expertise, it's essential to assess their skills in testing and evaluation. Focusing on key skills can provide valuable insights, and core competencies like testing are particularly important to evaluate.
Testing is a vital part of the software development process, ensuring that applications work as expected and are free of bugs. Be ready to discuss your experience with testing in Next.js, including unit testing, integration testing, and end-to-end testing.
A mix of practical tests and targeted questions helps find the right fit for your team, and a Next.js skills test can quickly identify top performers, saving time in your hiring process.
To assess a candidate's Next.js expertise, use the questions from this post to dive deeper into their Next.js expertise and problem-solving abilities after the test.
Consider reading: Testing-library/jest-dom How to Test with Next Js
Best Practices and Strategies
To ace a Next.js interview, it's essential to have a solid understanding of the framework's fundamental concepts. This includes a thorough grasp of Next.js's features, server-side rendering, and static site generation.
Mastering Next.js's data fetching methods and routing capabilities will greatly enhance your ability to build robust and efficient web applications. To achieve this, focus on understanding core language concepts, such as Next.js syntax, server-side rendering (SSR), and static site generation (SSG).
Proficiency in Next.js data fetching methods like getServerSideProps, getStaticProps, and getStaticPaths is crucial for managing routing with Next.js's file-based routing system. Familiarity with Next.js core features, commonly used plugins, and tools such as the Next.js CLI for project setup and Vercel for deployment is also vital.
Building and contributing to Next.js projects, solving real-world problems, and showcasing hands-on experience with components, data fetching, and Next.js's ecosystem is key to demonstrating practical experience. Writing unit and integration tests with tools like Jest and React Testing Library, and applying debugging techniques specific to Next.js applications, will also help you stand out.
Here are some key areas to focus on when preparing for a Next.js interview:
Typescript and Code Splitting
In Next.js, code splitting is handled automatically, splitting each page into its own bundle when you create a new page in the pages directory.
This means that only the necessary JavaScript for each page is loaded as the user navigates to different pages, making the initial load faster.
You can also manually implement dynamic imports using the next/dynamic module for components that you want to load lazily.
Using TypeScript
To use TypeScript with Next.js, you first install TypeScript and the necessary type definitions by running npm install --save-dev typescript @types/react @types/node.
Creating a tsconfig.json file in your project's root directory is as simple as running npx tsc --init. Next.js will automatically configure your development server based on your TypeScript setup.
You can start renaming your .js or .jsx files to .ts or .tsx, respectively, and begin writing your components and logic using TypeScript.
If any configuration tweaks are needed, you can adjust the tsconfig.json file to fine-tune your TypeScript setup.
See what others are reading: Convert Next Js Project to Typescript
How Code Splitting Works
Code splitting is an essential technique for optimizing the performance of your application. In Next.js, it's handled automatically, splitting each page into its own bundle.
This means that only the necessary JavaScript for the current page is loaded when a user navigates to a different page, making the initial load faster. As a result, your application feels snappier and more responsive.
You can also manually implement dynamic imports using the next/dynamic module for components that you want to load lazily. This ensures that parts of your application are only loaded when they are actually needed, instead of bundling everything together.
Sources
- https://mentorcruise.com/questions/nextjs/
- https://codeinterview.io/interview-questions/next-js-questions-answers
- https://www.adaface.com/blog/next-js-framework-interview-questions/
- https://moldstud.com/articles/p-what-are-the-common-interview-questions-for-nextjs-developers
- https://www.jobi.ai/nextjs-interview-questions
Featured Images: pexels.com